# Invoices
With the Apirone API, you can issue invoices that allow for seamless payments made by customers in crypto Accounts. In the requests, a customer can see all the invoice details, as well as check its status, callback information and history. The invoice can contain any data parameters which the customer would like to see in the invoice. These are predefined fields that the Apirone service added to the invoice, for example, title, merchant, store URL, goods or service items subtotal price, price, goods or service items, extras, etc.
# Create Invoice
Generates an invoice. Customer should have the Apirone account to create invoice.
# Request
- HTTP Method:
POST
- Content Type:
application/json
- URL:
https://apirone.com/api/v2/accounts/{account}/invoices
# Request example:
curl -X POST 'https://apirone.com/api/v2/accounts/apr-e729d9982f079fa86b10a0e3aa6ff37b/invoices' \
-H 'Content-Type: application/json' \
-d '{
"amount": 555000,
"currency": "btc",
"lifetime": 3600,
"callback_url": "http://example.com",
"user-data": {
"title": "Invoice for shop",
"merchant": "SHOP",
"url": "http://exampleshop.com",
"price": "$170",
"sub-price": "$160",
"items": [
{
"name": "box",
"cost": "$10",
"qty": 10,
"total": "$100"
},
{
"name": "hat",
"cost": "$5",
"qty": 10,
"total": "$50"
},
{
"name": "cap",
"cost": "$1",
"qty": 10,
"total": "$10"
}
],
"extras": [
{
"name": "Shipping",
"price": "$5"
},
{
"name": "Tax name",
"price": "$5"
}
]
},
"linkback": "http://linkback.com"
}'
Parameter | Type | Description | Required |
---|---|---|---|
currency | string | Currency type (btc, ltc, bch, doge, trx, usdt@trx) supported by service | ✓ |
amount | integer | Amount for the checkout in the selected currency of the invoice object. Also you may create invoices without fixed amount. The amount is indicated in minor units (e.g. 0.005 BTC shall be specified as 500,000, e.g. for usdt@trx: 50 usd shall be specified as 50,000000) | |
lifetime | integer | Duration of invoice validity (indicated in seconds) | |
expire | string | Invoice expiration time in ISO-8601 (opens new window) format, for example, 2022-02-22T09:00:30. If both parameters are specified: lifetime and expire , then the parameter expire will take precedence | |
callback-url | string | Callback URL used for invoice status updates notifications. More information about invoice callback see here | |
user-data | object | You can specify any data that you would like to see in the invoice. And there are some fields used for invoice representation, such as merchant, url, price, etc. | |
linkback | string | The customer will be redirected to this URL after the payment is completed |
Object user-data
consists of:
Parameter | Type | Description |
---|---|---|
title | string | Invoice title |
merchant | string | Merchant name |
url | string | Merchant url |
price | string | Displays the total price in fiat |
sub-price | string | Displays amount in fiat before adding discount, tax or shipping charges |
items | array | Consists of objects with predefined fields: name, cost, qty (quantity), total |
extras | array | Additional elements on an invoice e.g fees, taxes or shipping price |
Array items
:
Parameter | Type | Description |
---|---|---|
name | string | The name of the invoiced product/service is displayed in the field |
cost | string | Displays the unit price of the product |
qty | integer | The quantity of products/services is displayed in this field |
total | string | The total fiat price of the invoiced product/service is displayed in this field |
Array extras
:
Parameter | Type | Description |
---|---|---|
name | string | Name of extra charges |
price | string | Cost value |
# Example of user-data
object
"user-data": {
"title": "Invoice for shop",
"merchant": "SHOP",
"url": "http://exampleshop.com",
"price": "$170",
"sub-price": "$160",
"items": [
{
"name": "box",
"cost": "$10",
"qty": 10,
"total": "$100"
},
{
"name": "hat",
"cost": "$5",
"qty": 10,
"total": "$50"
},
{
"name": "cap",
"cost": "$1",
"qty": 10,
"total": "$10"
}
],
"extras": [
{
"name": "Shipping",
"price": "$5"
},
{
"name": "Tax name",
"price": "$5"
}
]
}
With each changing invoice status the Apirone service notifies the specified callback URL.
At the time expiration, the invoice status switches to expired
, and our service sends a callback. Subsequent actions with this invoice do not call a callback. In this case, all the funds received at the invoice address are processed the same way as with a valid invoice.
# Success Response Reference
- HTTP Status Code:
200
- Content Type:
application/json
Parameter | Type | Description |
---|---|---|
invoice | string | Invoice Identifier |
created | string | Invoice creation date. Contains the full date in ISO-8601 (opens new window) format, for example, 2022-02-22T09:00:30 |
account | string | Account Identifier |
currency | string | Currency type |
amount | integer | Amount in the selected currency |
expire | string | Invoice expiration time in ISO-8601 (opens new window) format, for example, 2022-02-22T09:00:30 |
address | string | The generated cryptocurrency address to receive a payment from a customer |
callback-url | string | Callback URL to receive data about the payment |
user-data | object | Some additional information about the invoice (includes following fields: title, merchant,url, price, sub-price, items, extras, etc.) |
history | array | Invoice status change history |
linkback | string | The customer will be redirected to this URL after the payment is completed |
status | string | Invoice status. More information see here |
invoice-url | string | Link to the invoice web view |
# Response example:
{
"invoice": "amr94MKUQCYAzR6c",
"created": "2022-04-27T12:02:49.375153",
"account": "apr-e729d9982f079fa86b10a0e3aa6ff37b",
"currency": "btc",
"amount": 555000,
"expire": "2022-04-27T13:02:49.375153",
"address": "3HfNiiSFfCJsvMD8joofyt3JCV9iy4N8xz",
"callback-url": "http://example.com",
"user-data": {
"title": "Invoice for shop",
"merchant": "SHOP",
"url": "http://exampleshop.com",
"price": "$170",
"sub-price": "$160",
"items": [
{
"name": "box",
"cost": "$10",
"qty": 10,
"total": "$100"
},
{
"name": "hat",
"cost": "$5",
"qty": 10,
"total": "$50"
},
{
"name": "cap",
"cost": "$1",
"qty": 10,
"total": "$10"
}
],
"extras": [
{
"name": "Shipping",
"price": "$5"
},
{
"name": "Tax name",
"price": "$5"
}
]
},
"linkback": "http://linkback.com",
"status": "created",
"invoice-url": "https://apirone.com/invoice?id=amr94MKUQCYAzR6c"
}
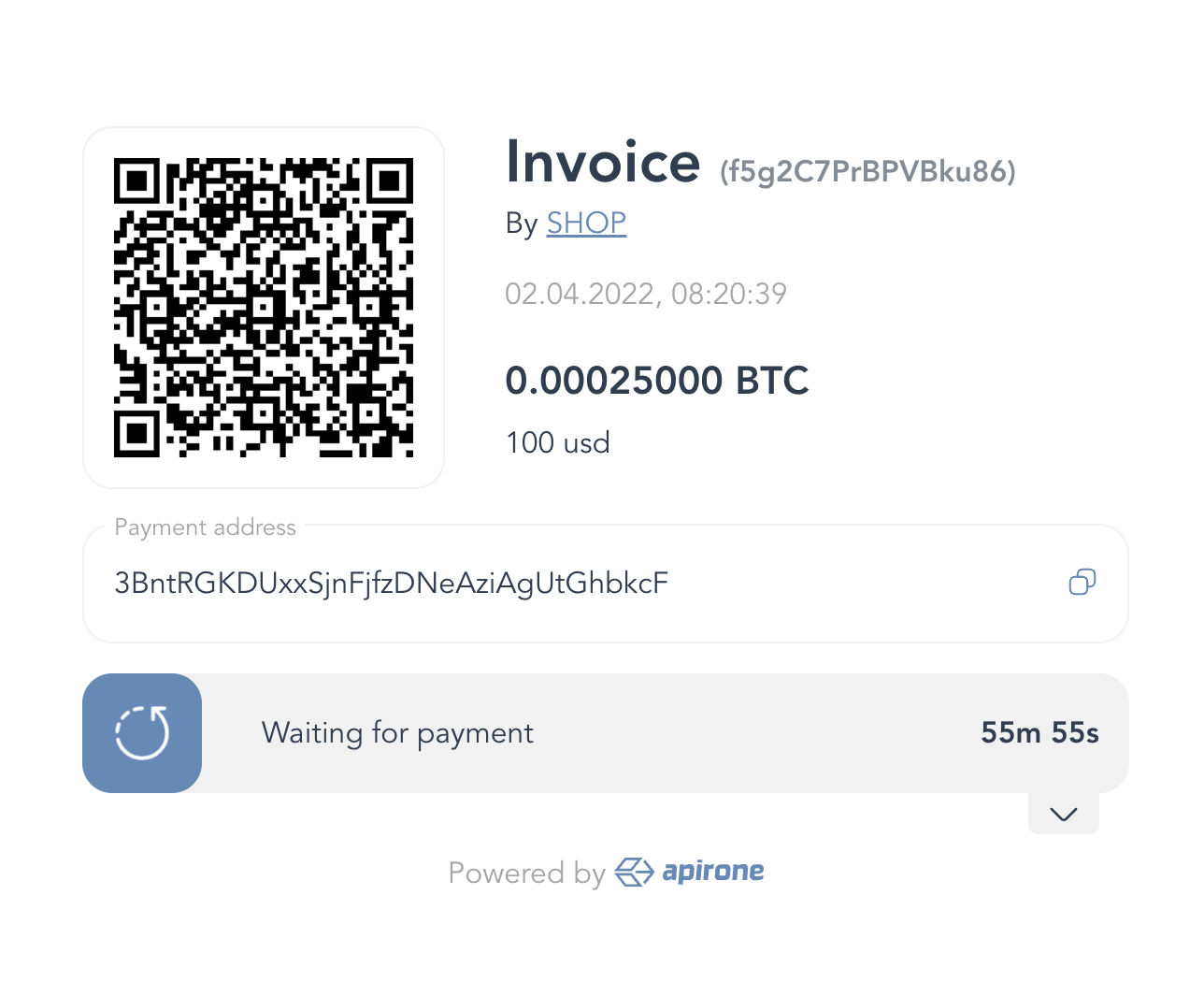
# Error response
- HTTP Status Code:
400
500
- Content Type:
application/json
Playground
# Public Invoice Info
Checks the invoice information and its status.
# Request
- HTTP Method:
GET
- URL:
https://apirone.com/api/v2/invoices/{invoice}
Parameter | Type | Description | Required |
---|---|---|---|
invoice | string | Invoice Identifier | ✓ |
# Request example
curl 'https://apirone.com/api/v2/invoices/amr94MKUQCYAzR6c'
# Success Response Reference
Parameter | Type | Description |
---|---|---|
invoice | string | Invoice Identifier |
created | string | Invoice creation date in ISO-8601 (opens new window) format (for example, 2022-02-22T09:00:30) |
currency | string | Currency type |
address | string | The generated cryptocurrency address to receive a payment from a customer |
expire | string | Invoice expiration time in ISO-8601 (opens new window) format (for example, 2022-02-22T09:00:30) |
amount | integer | Amount for the checkout in the selected currency |
user-data | object | Some specified data in the invoice |
status | string | Invoice status |
history | array | Invoice status change history |
linkback | string | The customer will be redirected to this URL after the payment is completed |
invoice-url | string | Link to the invoice web view |
Object history
consists on:
Type | Description | |
---|---|---|
date | string | Invoice status change date |
status | string | Invoice status |
txid | string | Identifier of the transaction in the blockchain |
amount | integer | Paid amount |
# Invoice Status
Type | Description |
---|---|
created | Initial state when the invoice is created in the account |
paid | As soon as the payment is received, the service compares the paid amount with the invoice requested amount. If the paid amount is equal to the amount expected, then the invoice is marked as paid |
partpaid | Status will be returned for cases when the customer partially paid for the invoice. When an invoice is expired with the ‘partially paid’ status, check the amount arrived. The difference in some Satoshi may be unimportant for you. Otherwise ,contact the payer to send the amount remained or cancel the order and refund the money |
overpaid | Status will be returned, if the payment from a customer exceeds the amount indicated in the invoice. This invoice is completed but you should contact the payer directly to refund the difference |
completed | When the block with the invoice's transaction is added to the blockchain, the transaction received the required number of confirmations, the invoice gets this status |
expired | The invoice has got the "expired" status, if the expiration date has arrived. But if the transaction has already been sent and stuck in the blockchain, the invoice will be closed after the payment confirmation |
# Response example
{
"invoice": "amr94MKUQCYAzR6c",
"created": "2022-04-27T12:02:49.375153",
"currency": "btc",
"address": "3HfNiiSFfCJsvMD8joofyt3JCV9iy4N8xz",
"expire": "2022-04-27T13:02:49.375153",
"amount": 555000,
"user-data": {
"title": "Invoice for shop",
"merchant": "SHOP",
"url": "http://exampleshop.com",
"price": "$170",
"sub-price": "$160",
"items": [
{
"name": "box",
"cost": "$10",
"qty": 10,
"total": "$100"
},
{
"name": "hat",
"cost": "$5",
"qty": 10,
"total": "$50"
},
{
"name": "cap",
"cost": "$1",
"qty": 10,
"total": "$10"
}
],
"extras": [
{
"name": "Shipping",
"price": "$5"
},
{
"name": "Tax name",
"price": "$5"
}
]
},
"status": "completed",
"history": [
{
"date": "2022-04-27T12:02:49.375153",
"status": "created"
},
{
"date": "2022-04-27T12:04:24.251921",
"status": "partpaid",
"txid": "26cfe85c09e22c423624fd23b2380bce626ea94db6fb5093bfa012e7407f25b1",
"amount": 190000
},
{
"date": "2022-04-27T12:05:25.241341",
"status": "paid",
"txid": "289c24a8804369c0afe9751d00aa0f19449cc05a1b00c37de078612b55821a72",
"amount": 365000
},
{
"date": "2022-04-27T12:08:25.050088",
"status": "completed"
}
],
"linkback": "http://linkback.com",
"invoice-url": "https://apirone.com/invoice?id=amr94MKUQCYAzR6c"
}
# Error response
- HTTP Status Code:
400
500
- Content Type:
application/json
Playground
# Private Invoice Info
The response contains information about the invoice including security-sensitive data (e.g. callback info). Authorization is required.
# Request
- HTTP Method:
GET
- URL:
https://apirone.com/api/v2/accounts/{account}/invoices/{invoice}
Parameter | Type | Description | Required |
---|---|---|---|
account | string | Account Identifier | ✓ |
invoice | string | Invoice Identifier | ✓ |
transfer-key | string | Key for accessing protected endpoints | * |
* transfer-key
is required unless access token is applied
# Request example
curl 'https://apirone.com/api/v2/accounts/apr-e729d9982f079fa86b10a0e3aa6ff37b/invoices/amr94MKUQCYAzR6c?transfer-key=82ookirnTwWNXXqFwdOQMVZIamt8s1uT'
# Success Response Reference
Parameter | Type | Description |
---|---|---|
account | string | Account Identifier |
invoice | string | Invoice Identifier |
created | string | Invoice creation date |
currency | string | Currency type |
amount | integer | Amount for the checkout in the specified currency |
expire | string | Invoice expiration time in ISO-8601 (opens new window) format (for example, 2022-02-22T09:00:30) |
address | string | The generated cryptocurrency address to receive a payment from a customer |
user-data | object | Some specified data in the invoice. More information about user-data object is here |
status | string | Invoice status |
history | array | Changes of the invoice status in history (contains change of the status date and the invoice status) |
callback-url | string | Callback URL |
linkback | string | The customer will be redirected to this URL after payment is completed |
invoice-url | string | Link to the invoice web view |
# Response example
{ "account": "apr-e729d9982f079fa86b10a0e3aa6ff37b",
"invoice": "amr94MKUQCYAzR6c",
"created": "2022-04-27T12:02:49.375153",
"currency": "btc",
"amount": 555000,
"expire": "2022-03-13T11:36:37.342134",
"address": "3HfNiiSFfCJsvMD8joofyt3JCV9iy4N8xz",
"user-data": {
"title": "Invoice for shop",
"merchant": "SHOP",
"url": "http://exampleshop.com",
"price": "$170",
"sub-price": "$160",
"items": [
{
"name": "box",
"cost": "$10",
"qty": 10,
"total": "$100"
},
{
"name": "hat",
"cost": "$5",
"qty": 10,
"total": "$50"
},
{
"name": "cap",
"cost": "$1",
"qty": 10,
"total": "$10"
}
],
"extras": [
{
"name": "Shipping",
"price": "$5"
},
{
"name": "Tax name",
"price": "$5"
}
]
},
"status": "completed",
"history": [
{
"date": "2022-04-27T12:02:49.375153",
"status": "created"
},
{
"date": "2022-04-27T12:04:24.251921",
"status": "partpaid",
"txid": "26cfe85c09e22c423624fd23b2380bce626ea94db6fb5093bfa012e7407f25b1",
"amount": 190000
},
{
"date": "2022-04-27T12:05:25.241341",
"status": "paid",
"txid": "289c24a8804369c0afe9751d00aa0f19449cc05a1b00c37de078612b55821a72",
"amount": 365000
},
{
"date": "2022-04-27T12:08:25.050088",
"status": "completed"
}
],
"callback-url": "https://example.com",
"linkback": "http://linkback.com",
"invoice-url": "https://apirone.com/invoice?id=amr94MKUQCYAzR6c"
}
# Error response
- HTTP Status Code:
400
500
- Content Type:
application/json
Playground
# Invoices List
Shows a list of all invoices in the account. Contains information about each invoice. Authorization is required.
# Request
- HTTP Method:
GET
- URL:
https://apirone.com/api/v2/accounts/{account}/invoices
Parameter | Type | Description | Required |
---|---|---|---|
account | string | Account Identifier | ✓ |
transfer-key | string | Key for accessing protected endpoints | * |
q | string | Filter items by specific criteria |
* transfer-key
is required unless access token is applied
The filter q
is assembled into a string by bare listing of the variables in the string, separated by commas. A colon is used as a separator between the parameter name and the value.
# Example of q
:
status:created,date_from:2022-06-12,date_to:2022-08-11
Can contain the following options:
Parameter | Description |
---|---|
status | Invoice status |
date_from , date_to | The date of invoice creation |
# Request example
curl 'https://apirone.com/api/v2/accounts/apr-e729d9982f079fa86b10a0e3aa6ff37b/invoices?q=status:created,date_from:2021-10-22,date_to:2021-12-21&transfer-key=82ookirnTwWNXXqFwdOQMVZIamt8s1uT'
# Success Response Reference
- HTTP Status Code:
200
- Content Type:
application/json
Parameter | Type | Description |
---|---|---|
account | string | Account Identifier |
date_from | string | Default value: the date when the account is created. Contains the full date, hours, minutes, seconds, decimal part of seconds (for example, 2021-01-22T23:00:30) |
date_to | string | Default value: today's date (for example, 2022-02-22T09:00:30) |
items | array | An array containing information about invoices |
pagination | array | Pagination info |
Element items
:
Parameter | Type | Description |
---|---|---|
invoice | string | Invoice Identifier |
created | string | Invoice creation date (for example, 2022-02-22T09:00:30). |
currency | string | Currency type |
amount | integer | The amount specified when creating the invoice. |
status | string | Invoice status. More information about the status see here |
Element pagination
:
Parameter | Type | Description |
---|---|---|
total | integer | Total number of items |
offset | integer | The sequence number of the element from which the counting starts. Default value: 0 |
limit | integer | Number of items displayed that request will return. Default value: 10 |
# Response example
{
"account": "apr-e729d9982f079fa86b10a0e3aa6ff37b",
"date_from": "2021-10-22T08:58:14.253612",
"date_to": "2021-12-21T07:16:07.847586",
"items": [
{
"invoice": "06xm4jZXj2QOCl7a",
"created": "2021-12-14T19:22:37.697770",
"currency": "btc",
"amount": 20000,
"status": "created"
},
{
"invoice": "1qR3v7JPQnSxM784",
"created": "2021-11-26T14:27:13.858791",
"currency": "btc",
"amount": 30000,
"status": "created"
}
],
"pagination":
{
"limit": 10,
"offset": 0,
"total": 3
}
}
# Error response
- HTTP Status Code:
400
500
- Content Type:
application/json